Para este ejemplo usaremos un archivo de texto sencillo con sólo tres líneas:
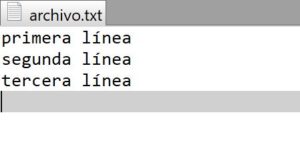
El código siguiente te permitirá leer el texto del archivo entero, usando System.IO.File.ReadAllText.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace LeerArchivoTxtCsharp { class Program { static void Main(string[] args) { string text = System.IO.File.ReadAllText(@"C:\ruta\archivo.txt"); System.Console.WriteLine("Contenido del archivo = {0}", text); Console.WriteLine("Presione cualquier tecla para salir."); System.Console.ReadKey(); } } }
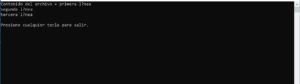
El resultado en consola es el siguiente:
Este segundo método te permitirá leer línea por línea con System.IO.File.ReadAllLines:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace LeerArchivoTxtCsharp { class Program { static void Main(string[] args) { string[] lines = System.IO.File.ReadAllLines(@"C:\ruta\archivo.txt"); System.Console.WriteLine("Contenido del archivo = "); foreach (string line in lines) { // Use a tab to indent each line of the file. Console.WriteLine("\t" + line); } Console.WriteLine("Presione cualquier tecla para salir."); System.Console.ReadKey(); } } }
El resultado es el siguiente:

En consola se aprecia que algunos caracteres no se despliegan correctamente, pero el contenido se lee correctamente, el problema sólo ocurre al imprimir los caracteres en consola.